개발보드인 아두이노 시리즈는 정말 실전 전자회로 자작품을 만드는데 전자공학에 기초가 없는 초보자도 접근하기 쉬운 개발키트이다. 프로그램으로 따지자면, BASIC 같은 것이리라.
 |
Arduino Uno |
그러나, 아두이노 우노 등 개발보드를 사용하다 보면 몇가지 고민거리가 생긴다. 하나는 잡다한 프로젝트를 연습삼아 개발해볼때 마다 아두이노 우노를 구매하기가 그렇다. 우노 보드의 정품가는 약 2만원대, 호환품은 약 5~6천원대이므로 간단한 여러가지 실험을 위해 여러개를 구매하는것은 좀 아깝고 부담도 된다. 필자는 지금도 그렇지만 초창기 여러가지 궁금한 것들을 테스트 하느라 벌려 놓은것이 많았고 그러한 이유로 Uno, Mega, Due 등 여러가지를 구매하여 사용하게 되었다. 이후로는 최대한 싸구려 호환보드를 구매하기 위해 알리익스프레스를 뒤적거리던 기억도 난다.
혹은, 매번 기존에 빵판에 만들어 놓은것을 해체하여 새로운 것을 시도하는것도 좀 무언가 아깝기도 하다. 기껏 시제품(?)을 만들어 놓고 해체하면 비싼 레고 구매해서 잘 만들어 놓고 다른 것 해보겠다고 뽀개는 것과 비슷하지 않은가.
또 하나의 문제점은 개발보드의 크기 자체에 있다. 간단한 시스템을 구현해 보려는데 가장 단순한 우노 보드만 봐도 손바닥 만한것이 소형화에 문제가 있고, 핵심 부품인 MCU(ATmega328p) 외에 나머지 별로 필요해 보이지 않는 것들까지 포함되는게 좀 거슬리기도 한다. 물론, 이런 경우를 위해 Arduino Pro Mini와 Arduino Nano와 같은 우노와 같은 성능의 소형 버전이 존재한다.
 |
Arduino Nano |
 |
Arduino Pro Mini |
Arduino Pro Mini의 경우 가장 소형이지만 스케치를 업로드 하기 위해서는 별도의 FTDI가 필요하다.
 |
FTDI232 |
Arduino Pro Mini를 봐도 내부에 전압 레귤레이터, 인디케이터 LED, 스위치 등 사실 경우에 따라 불필요한 소자들이 여전히 존재한다. MCU만 사용할 수 있으면 개발 테스트 보드에 직접 통합이 가능하므로 소형화에 가장 유리할 것이다. 그렇다면, 그냥 핵심 칩(MCU)만 사용할 수는 없을까?
물론 가능하다, 다만 그렇게 사용하기에는 몇가지 제약이 따른다. 여기서는 다루기 쉬운 DIP 타입을 기준으로 이야기 해보자. (SMD를 잘 다룰 수 있다면 SMD 타입을 사용할 수도 있겠다.)
 |
ATmega328p DIP type |
우선 DIP 형태의 ATmega328p(이하 328)는 만능기판에 사용할 수 있으니 논하기가 적합한것 같다. 그럼, 외형적인 이야기는 이만하고, MCU를 독립적인 형태로 사용하기 위한 보다 중요한 문제를 이야기 해보도록 하자.
우선 MCU가 동작하기 위해서는 기본적으로 외부 전원이 필요하다.
 |
ATmega328p Pinout |
328의 전원은 두곳이다. 핀번호 7, 8번과 22, 21번에서 328의 구동전압으로 전원을 인가한다. 전원을 넣는것 만으로도 328은 이미 동작을 시작할 수도 있다. 다만, 동작이 가능하려면 외부에서 클럭 신호가 주어져야 한다. 그 부분은 XTAL이라고 적혀있는 핀번호 9, 10번에 해당한다.
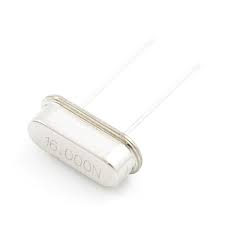 |
Crystal |
전원과 crystal + capacitor 세트로 이루어진 외부 클럭이면 328은 자체적으로 구동될 수 있다. 하지만, 이마저도 제거할 수 있는데, 그것은 328은 자체 발진기를 내부에 포함하고 있기 때문이다. 그런데 왜 외부 클럭을 구지 사용할까? 그것은 성능(정교함)의 차이 때문이라고 할 수 있을 것 같은데, 내부 클럭을 사용 시에는 328이 우노에서 기본 설정속도인 16Mhz를 사용할 수 없고 최대 8Mhz로 동작하기 때문이다.
16Mhz를 사용하지 않으면 되잖아? 맞는 말이다. 보통 IoT 구현을 위한 센서류를 다루다 보면, MCU의 역할이 연산의 속도 보다는 로직 구현이 더 중요하기 때문에, 빠르면 좋겠지만 8Mhz의 속도가 충분한 경우가 많다. 물론 로직의 구현이라고 하더라도, 센서와의 연동 과정에서 클럭의 정교함이 필요한 경우에는 무조건 빠른게 좋다. 왜냐면 타이머는 클럭에 맞추어 돌아가기 때문이다.
타이머 분주에 관련하여 다음 글을 읽어보기를 바란다:
하지만 오늘 우리의 가치는 소형화에 있다. 내장 발진기를 사용하는것으로 하고 다음 이야기를 진행해보도록 하자.
내장 발진기를 사용하게 되면 더 이상 328에 추가적으로 연계할 부품은 없다.
이제 이 상태에서 어떻게 프로그램(스케치)을 올리게 되는지 이야기를 해보자. 이 부분은 [2부] 에서 다루도록 하겠다.
아두이노의 편리함은 MCU에 대한 복잡한 설정부분을 감추고 프로그래밍에 필요한 주변장치를 필요없게 해주는 부트로더 덕분이 아닐까 한다. 다음에 바로 그 이야기를 해보도록 하자.
예고: 아두이노 최소화로 사용하기 [2부] 부트로더